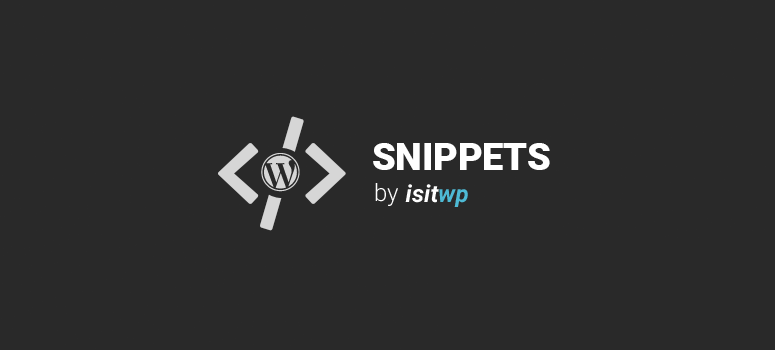
Are you looking for a way to track post views without a plugin using post meta? While there’s probably a plugin for this, we have created a quick code snippet that you can use to track post views without a plugin using post meta in WordPress.
Instructions:
Add this code to your theme’s functions.php file or in a site-specific plugin:
function getPostViews($postID){ $count_key = 'post_views_count'; $count = get_post_meta($postID, $count_key, true); if($count==''){ delete_post_meta($postID, $count_key); add_post_meta($postID, $count_key, '0'); return "0 View"; } return $count.' Views'; } function setPostViews($postID) { $count_key = 'post_views_count'; $count = get_post_meta($postID, $count_key, true); if($count==''){ $count = 0; delete_post_meta($postID, $count_key); add_post_meta($postID, $count_key, '0'); }else{ $count++; update_post_meta($postID, $count_key, $count); } } // Remove issues with prefetching adding extra views remove_action( 'wp_head', 'adjacent_posts_rel_link_wp_head', 10, 0);
Optionally add this code as well to a column in the WordPress admin that displays the post views:
// Add to a column in WP-Admin add_filter('manage_posts_columns', 'posts_column_views'); add_action('manage_posts_custom_column', 'posts_custom_column_views',5,2); function posts_column_views($defaults){ $defaults['post_views'] = __('Views'); return $defaults; } function posts_custom_column_views($column_name, $id){ if($column_name === 'post_views'){ echo getPostViews(get_the_ID()); } }
This part of the tracking views code will set the post views. Just place this code below within the single.php file inside the WordPress Loop.
<?php setPostViews(get_the_ID()); ?>
Note about fragment caching: If you are using a caching plugin like W3 Total Cache, the method above to set views will not work as the setPostViews()
function would never run. However, W3 Total Cache has a feature called fragment caching. Instead of the above, use the following so the setPostViews()
will run just fine and will track all your post views even when you have caching enabled.
<!-- mfunc setPostViews(get_the_ID()); --><!-- /mfunc -->
The code below is optional. Use this code if you would like to display the number of views within your posts. Place this code within the Loop.
<?php echo getPostViews(get_the_ID()); ?>
Note: If this is your first time adding code snippets in WordPress, then please refer to our guide on how to properly copy / paste code snippets in WordPress, so you don’t accidentally break your site.
If you liked this code snippet, please consider checking out our other articles on the site like: 10 best WordPress testimonial plugins and how to Set Up Author Tracking in WordPress with Google Analytics.
Hi,
Can I do like fake views count + real count?
It means I can set starting value manually (because I want my new published post to show a value like 3,429 views in the first minute) so that my post will look like it has a high amount of post views, of course, combined with real count also.
If yes, where to set the fake view count base on the code?
What would be the meta key for this to call it in a query?
‘post_views_count’ is the meta key.
Hi is it possible to make somehow the number of views display only if it’s more than 1000?
You could use this code when displaying the comment count:
if (getPostViews(get_the_ID()) >= 1000) {
echo getPostViews(get_the_ID());
}
Is there a way to exclude admin views from the count?
You may want to use something like this code to conditionally count views: https://www.isitwp.com/check-if-user-is-logged-in/
Hey Debjit Saha i can’t able to find single.php folder in my template, where should i paste the code?
The main loop for single posts may be in another template file. You may want to check for a post.php file or a similarly named file.
Thank you for this code. it worked perfectly.
Nice snippet.
How can I reset the counts and start counting again?
If you know the post id of the posts you want then this should work.
UPDATE yourDatabaseName.wp_postmeta
set meta_value = 0
where meta_key = ‘post_views_count’ and post_id = 1934
Hello, very good your post.
My question is how to bring the data from Google Analytics to show the number of views of Posts and Pages?
Do you have a Post or a link on how to implement this?
Sadly we don’t have a current recommendation for this exactly. That said, you may want to check out: https://www.monsterinsights.com/how-to-check-stats-for-individual-wordpress-posts-and-pages/
Any google analytics plugin that can pull view data and display in wordpress?
There are plenty… You can check out our complete list of best Google Analytics plugin.
The codes are not working for me.I have bbpress topic,means post type is “topic”. But when i checked meta_key is genearting in backend. But meta values are not updating on each visits
Great articles, it work fine on my page
my question is, does the count will just start after implementing that code ?
because all my old page showing 0 or 1 views while actually it already got thousands views.
Yes, the counting doesn’t start until the plugin is active. Any views before this were not recorded by this plugin.
Thanks a lot – works great!
I’ve ‘improved’ the function get_post_views slightly so it will return the proper text depending on the number of views. Here is my version:
function get_post_views($postID) {
$count_key = ‘post_views_count’;
$count = get_post_meta($postID, $count_key, true);
if($count==”){
delete_post_meta($postID, $count_key);
add_post_meta($postID, $count_key, ‘0’);
return “0 Views”;
}
if ($count==’1′) {
return “1 View”;
}
return $count.’ Views’;
}
Maybe you like to adopt this version.
Thanks for sharing! 🙂
thanks.
This has helped me a lot, thank you very much!
Great! Works like charm… I only wanted to view it in the admin area and just the first two codes do the trick!
Hi,
Thanks for the code, it works like a charm without cache enabled…
But, how can I make it work with wp rocket?
Please…
Anyone know how to format or truncate the numbers? So instead of 4445 views, it’s 4,445 or 4.5K ?
You may want to use PHP functions like round() and number_format() for this use case.
Hi, somehow my code runs fine but it is doing +2 increment each time, the views go 0, 2, 4, 6. Which has been annoying please help. I placed the code as well as the show post views portion both in a function that has a action after content. The counter works, just that its in multiples of 2.
You may want to check to make sure that this function, setPostViews(get_the_ID()), is only added once. Which hook are you using?
wil the work in lightspeed cache?
Code appearing on home page
Opps sorry, please disregard my previous comment. I realized I was calling the setPostViews function multiple times. My bad….
After fixing this, I am only seeing the view count go up +2 anytime I refresh the single blog post.
Does anyone have a fix for this? I don’t imagine this will be much of a problem considering that most users wont actually be refreshing the page, though it would be nice to have an actual count number to display..
Thanks for the code 🙂
How to making a random views count to (600,900)
please help..
how do i stop auto increase count on page refresh?
Hey, really great tips. Thanks for sharing with us. I am using plugin and google analytics to track my page views.
Hi,
I want to show the count inside meta class, my meta code is:
how to print the counter between the meta like author, date, catagories?