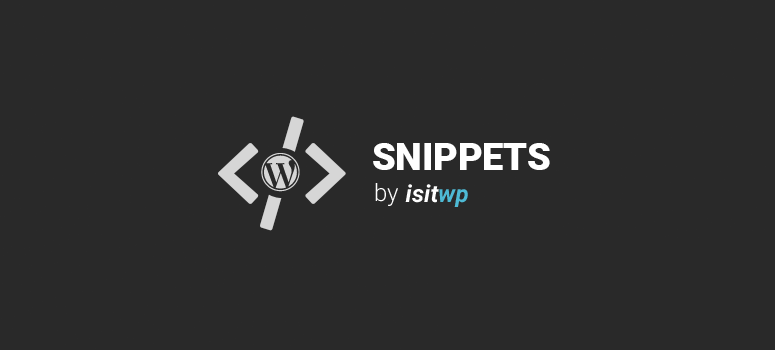
Are you looking for a way to track post views without a plugin using post meta? While there’s probably a plugin for this, we have created a quick code snippet that you can use to track post views without a plugin using post meta in WordPress.
Instructions:
Add this code to your theme’s functions.php file or in a site-specific plugin:
function getPostViews($postID){ $count_key = 'post_views_count'; $count = get_post_meta($postID, $count_key, true); if($count==''){ delete_post_meta($postID, $count_key); add_post_meta($postID, $count_key, '0'); return "0 View"; } return $count.' Views'; } function setPostViews($postID) { $count_key = 'post_views_count'; $count = get_post_meta($postID, $count_key, true); if($count==''){ $count = 0; delete_post_meta($postID, $count_key); add_post_meta($postID, $count_key, '0'); }else{ $count++; update_post_meta($postID, $count_key, $count); } } // Remove issues with prefetching adding extra views remove_action( 'wp_head', 'adjacent_posts_rel_link_wp_head', 10, 0);
Optionally add this code as well to a column in the WordPress admin that displays the post views:
// Add to a column in WP-Admin add_filter('manage_posts_columns', 'posts_column_views'); add_action('manage_posts_custom_column', 'posts_custom_column_views',5,2); function posts_column_views($defaults){ $defaults['post_views'] = __('Views'); return $defaults; } function posts_custom_column_views($column_name, $id){ if($column_name === 'post_views'){ echo getPostViews(get_the_ID()); } }
This part of the tracking views code will set the post views. Just place this code below within the single.php file inside the WordPress Loop.
<?php setPostViews(get_the_ID()); ?>
Note about fragment caching: If you are using a caching plugin like W3 Total Cache, the method above to set views will not work as the setPostViews()
function would never run. However, W3 Total Cache has a feature called fragment caching. Instead of the above, use the following so the setPostViews()
will run just fine and will track all your post views even when you have caching enabled.
<!-- mfunc setPostViews(get_the_ID()); --><!-- /mfunc -->
The code below is optional. Use this code if you would like to display the number of views within your posts. Place this code within the Loop.
<?php echo getPostViews(get_the_ID()); ?>
Note: If this is your first time adding code snippets in WordPress, then please refer to our guide on how to properly copy / paste code snippets in WordPress, so you don’t accidentally break your site.
If you liked this code snippet, please consider checking out our other articles on the site like: 10 best WordPress testimonial plugins and how to Set Up Author Tracking in WordPress with Google Analytics.
Hi, looking for query to remove posts without views, any ideas ? thanks 🙂
Kevin, Thanks for this nice snippet.
How will this work for a site with a very large traffic volume? I was looking into a post view plugin that is very popular (can’t remember the name of it now), and that was a concern mentioned in the comments.
It works fine with new versions of WP. Make sure you have things setup correctly.
Hi, there any way to reset the counter to zero views?
Hi Cyndy everything is saved as meta data so you can just go into the post and change the value from whatever it is to zero.
Kevin, thanks
your tutorial very useful!!
Hi Cyndy, Hi Kevin.
Cool code.
I can’t find the exact place where this meta data is stored for each post to change it.
Inside the post (From Dashboard->Post->Edit) there is only the content of the post, no meta data.
Thanks.
Hi Kevin!
I used your snippet for some time now and I’m happy, but in the last weeks something happened and it stopped counting all the views. I know this because i see 500 views from google analytics, yet the snippet shows only 18 views? I’m using the exact code you presented here, no modifications. I just changed a count last month and since then everything stopped working.
Normally this would be because you have installed a new plugin or something along that lines. I have tested this snippet on new versions of wordpress and it should work just fine. Do you happen to have a cache plugin running on your site, like w3 total cache?
I do have w3 installed. how is it affecting the snippet? it worked until now with it side by side, why is it causing problems now? I cleaned the cache many times and still nothing. ughhhh!
because it would not be calling the setPost function since things are cached, send me an email using the contact form and ill send you and updated version that will solve the problem.
can you share here?
Is there any way for the views count to be reset every day in order to show just the number of views for the current day?
Hi Pere,
Yes absolutely, but you would have to change a fair amount of code to achieve this. Unfortunately not just a simple change,
This is exactly what I am looking for. Is there any place where I can get this information?
I need to implement a Trending Section Page for a customer of mine and I am a bit stuck with this at the moment. It seems I can’t get any information about it around the web.
Any help would be very much appreciated!
Hi Pere, did you find anything out about it?
Is there anyway to get this to only register unique views? Bashing f5 on my keyboard is cranking up the numbers.
Hi Mark,
Yes but not easily with this snippet. You could do some cookie checking, or tracking of ip addresses for better but not perfect unique views. Or even require users to be logged in and track on a users by user basis.
$count = 0; in setPostViews() on line #15 should be removed, it doesn’t really do anything 😉
Hands down the quickest snippet to implement
I’m using it for several frontend notification methods, great work
You rock!
thank’s this tutorial
Where is the count stored? Is it in a database?
Wanted to know if it’s possible to change the values.
Works well for me on latest version of wordpress, thanks.
for me it is counting twice…
Hi Andrew, is likely prefetching, or the setViews is added more than once. To solve the one issue try adding this. Note the code above.
// Remove issues with prefetching adding extra views
remove_action( ‘wp_head’, ‘adjacent_posts_rel_link_wp_head’, 10, 0);
Hi, this seems to stop working in WP 4.0. Can anyone confirm or provide fix? thx u!
Have you heard anything on this? I just upgraded to 4.0, and would like to use this.
Same here.
I’m using 4.0 and nothing wrong 🙂
Wow. This saved me time. Very nice dude thank you.
Great snippet but still incrementing on every post.
Hello!
Sorry for bad english, I’m using a translator. I’m from Brazil.
I have a site that already has several views on the post, as I do not lose these views? Is to update the database? I use this plugin: WP-PostViews
Would you happen to help me in styling the echo output. I want to put the views count on my loop and I accomplished that perfectly but how do I add a css to the output for the text? Any ideas on that. Thank You
Hi Randy you can do the following by adding a div around the php function. i added a sample on pastebin
http://pastebin.com/ThLasSJB
I tried that right now and its not working.
I tried that right now and its not working.
I tried that right now and its not working.
I tried that right now and its not working.
I tried that right now and its not working.
I tried that right now and its not working.
Hi Randy,
is the snippet displaying the number of views? if so it has something to do with your css or html as copying that snippet and placing it within your single post loop should work fine. You just have to define some styles to apply.
Would you happen to help me in styling the echo output. I want to put the views count on my loop and I accomplished that perfectly but how do I add a css to the output for the text? Any ideas on that. Thank You
Would you happen to help me in styling the echo output. I want to put the views count on my loop and I accomplished that perfectly but how do I add a css to the output for the text? Any ideas on that. Thank You
Would you happen to help me in styling the echo output. I want to put the views count on my loop and I accomplished that perfectly but how do I add a css to the output for the text? Any ideas on that. Thank You
Would you happen to help me in styling the echo output. I want to put the views count on my loop and I accomplished that perfectly but how do I add a css to the output for the text? Any ideas on that. Thank You
Would you happen to help me in styling the echo output. I want to put the views count on my loop and I accomplished that perfectly but how do I add a css to the output for the text? Any ideas on that. Thank You
Would you happen to help me in styling the echo output. I want to put the views count on my loop and I accomplished that perfectly but how do I add a css to the output for the text? Any ideas on that. Thank You
Would you happen to help me in styling the echo output. I want to put the views count on my loop and I accomplished that perfectly but how do I add a css to the output for the text? Any ideas on that. Thank You