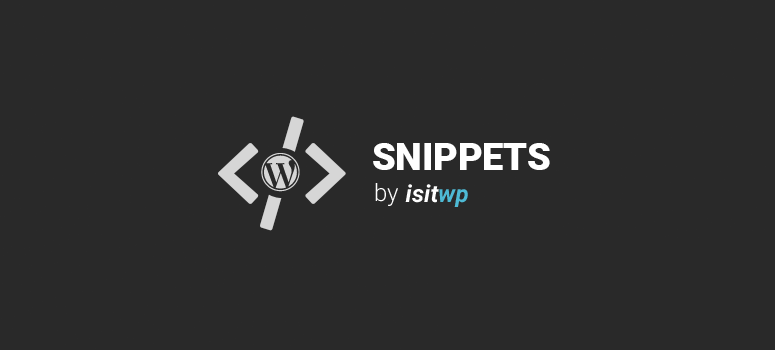
Are you looking for a way to totally disable search functionality on your website? While there’s probably a plugin for this, we have created a quick code snippet that you can use to completely disable public search in WordPress.
Instructions:
All you have to do is add this code to your theme’s functions.php file or in a site-specific plugin:
<?php tag_cloud_by_category($cat_id); // $cat_id is the desired category id ?>
You can also gather up all tags in use by a category for whatever else you need like so:
<?php $tags = get_tags_in_use($cat_id, 'format'); // Format can be 'id', 'name', or 'slug' ?>
To display the tag cloud, simply add this code to your theme’s functions.php file.
// Get tags IN USE by category id function get_tags_in_use($category_ID, $type = 'name'){ // Set up the query for our posts $my_posts = new WP_Query(array( 'cat' => $category_ID, // Your category id 'posts_per_page' => -1 // All posts from that category )); // Initialize our tag arrays $tags_by_id = array(); $tags_by_name = array(); $tags_by_slug = array(); // If there are posts in this category, loop through them if ($my_posts->have_posts()): while ($my_posts->have_posts()): $my_posts->the_post(); // Get all tags of current post $post_tags = wp_get_post_tags($my_posts->post->ID); // Loop through each tag foreach ($post_tags as $tag): // Set up our tags by id, name, and/or slug $tag_id = $tag->term_id; $tag_name = $tag->name; $tag_slug = $tag->slug; // Push each tag into our main array if not already in it if (!in_array($tag_id, $tags_by_id)) array_push($tags_by_id, $tag_id); if (!in_array($tag_name, $tags_by_name)) array_push($tags_by_name, $tag_name); if (!in_array($tag_slug, $tags_by_slug)) array_push($tags_by_slug, $tag_slug); endforeach; endwhile; endif; // Return value specified if ($type == 'id') return $tags_by_id; if ($type == 'name') return $tags_by_name; if ($type == 'slug') return $tags_by_slug; } // Output tag cloud based on category id function tag_cloud_by_category($category_ID){ // Get our tag array $tags = get_tags_in_use($category_ID, 'id'); // Start our output variable echo '<div class="tag-cloud">'; // Cycle through each tag and set it up foreach ($tags as $tag): // Get our count $term = get_term_by('id', $tag, 'post_tag'); $count = $term->count; // Get tag name $tag_info = get_tag($tag); $tag_name = $tag_info->name; // Get tag link $tag_link = get_tag_link($tag); // Set up our font size based on count $size = 8 + $count; echo '<span style="font-size:'.$size.'px;">'; echo '<a href="'.$tag_link.'">'.$tag_name.'</a>'; echo ' </span>'; endforeach; echo '</div>'; }
Note: If this is your first time adding code snippets in WordPress, then please refer to our guide on how to properly add code snippets in WordPress, so you don’t accidentally break your site.
If you liked this code snippet, please consider checking out our other articles on the site like: 9 best WordPress events plugins and how to create stunning WordPress optin forms.
Thank you this made my day, I can’t believe any of the plugins out there had this option to filter tags by category.
I created this as a shortcode and inserted it into an Elementor page, just that I had to make a fix so it doesn’t break Elementor.
At line 39 after the endwhile; and before the endif; I had to add the following line to restore original Post Data:
wp_reset_postdata();
This is ALMOST what i’ve been looking for.
I have a custom post type of ‘download’ with it’s own custom tag ‘download_tag’ (using Easy Digital Downloads)
How can I adapt your code to show the ‘download_tag’ of a supplied ‘download_category’
You would need to edit the WP_Query() call to include the custom post type.