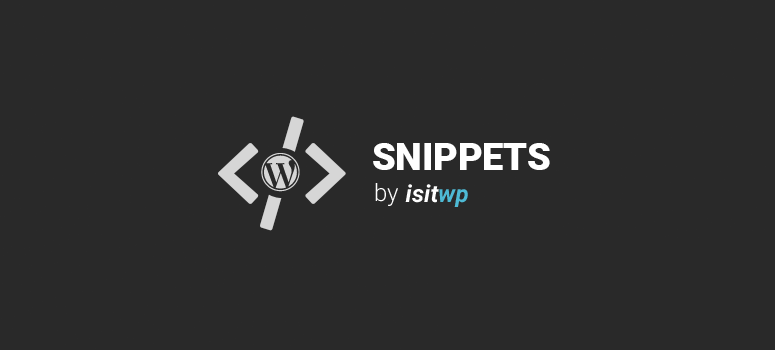
Are you looking for a way to add breadcrumbs and display them in WordPress? While there’s probably a plugin for this, we have created a quick code snippet that you can use to add breadcrumbs in WordPress.
Instructions:
All you have to do is add this code to your theme’s functions.php file or in a site-specific plugin:
function dimox_breadcrumbs() { $delimiter = '»'; $name = 'Home'; //text for the 'Home' link $currentBefore = '<span class="current">'; $currentAfter = '</span>'; if ( !is_home() && !is_front_page() || is_paged() ) { echo '<div id="crumbs">'; global $post; $home = get_bloginfo('url'); echo '<a href="' . $home . '">' . $name . '</a> ' . $delimiter . ' '; if ( is_category() ) { global $wp_query; $cat_obj = $wp_query->get_queried_object(); $thisCat = $cat_obj->term_id; $thisCat = get_category($thisCat); $parentCat = get_category($thisCat->parent); if ($thisCat->parent != 0) echo(get_category_parents($parentCat, TRUE, ' ' . $delimiter . ' ')); echo $currentBefore . 'Archive by category ''; single_cat_title(); echo ''' . $currentAfter; } elseif ( is_day() ) { echo '<a href="' . get_year_link(get_the_time('Y')) . '">' . get_the_time('Y') . '</a> ' . $delimiter . ' '; echo '<a href="' . get_month_link(get_the_time('Y'),get_the_time('m')) . '">' . get_the_time('F') . '</a> ' . $delimiter . ' '; echo $currentBefore . get_the_time('d') . $currentAfter; } elseif ( is_month() ) { echo '<a href="' . get_year_link(get_the_time('Y')) . '">' . get_the_time('Y') . '</a> ' . $delimiter . ' '; echo $currentBefore . get_the_time('F') . $currentAfter; } elseif ( is_year() ) { echo $currentBefore . get_the_time('Y') . $currentAfter; } elseif ( is_single() && !is_attachment() ) { $cat = get_the_category(); $cat = $cat[0]; echo get_category_parents($cat, TRUE, ' ' . $delimiter . ' '); echo $currentBefore; the_title(); echo $currentAfter; } elseif ( is_attachment() ) { $parent = get_post($post->post_parent); $cat = get_the_category($parent->ID); $cat = $cat[0]; echo get_category_parents($cat, TRUE, ' ' . $delimiter . ' '); echo '<a href="' . get_permalink($parent) . '">' . $parent->post_title . '</a> ' . $delimiter . ' '; echo $currentBefore; the_title(); echo $currentAfter; } elseif ( is_page() && !$post->post_parent ) { echo $currentBefore; the_title(); echo $currentAfter; } elseif ( is_page() && $post->post_parent ) { $parent_id = $post->post_parent; $breadcrumbs = array(); while ($parent_id) { $page = get_page($parent_id); $breadcrumbs[] = '<a href="' . get_permalink($page->ID) . '">' . get_the_title($page->ID) . '</a>'; $parent_id = $page->post_parent; } $breadcrumbs = array_reverse($breadcrumbs); foreach ($breadcrumbs as $crumb) echo $crumb . ' ' . $delimiter . ' '; echo $currentBefore; the_title(); echo $currentAfter; } elseif ( is_search() ) { echo $currentBefore . 'Search results for '' . get_search_query() . ''' . $currentAfter; } elseif ( is_tag() ) { echo $currentBefore . 'Posts tagged ''; single_tag_title(); echo ''' . $currentAfter; } elseif ( is_author() ) { global $author; $userdata = get_userdata($author); echo $currentBefore . 'Articles posted by ' . $userdata->display_name . $currentAfter; } elseif ( is_404() ) { echo $currentBefore . 'Error 404' . $currentAfter; } if ( get_query_var('paged') ) { if ( is_category() || is_day() || is_month() || is_year() || is_search() || is_tag() || is_author() ) echo ' ('; echo __('Page') . ' ' . get_query_var('paged'); if ( is_category() || is_day() || is_month() || is_year() || is_search() || is_tag() || is_author() ) echo ')'; } echo '</div>'; } }
Add this code to your theme’s template file where you want to show the breadcrumbs.
<?php if (function_exists('dimox_breadcrumbs')) dimox_breadcrumbs(); ?>
Alternatively, you can you this shorter, similar snippet. Add this code to your theme’s functions.php file or in a site-specific plugin:
function the_breadcrumb() { echo '<ul id="crumbs">'; if (!is_home()) { echo '<li><a href="'; echo get_option('home'); echo '">'; echo 'Home'; echo "</a></li>"; if (is_category() || is_single()) { echo '<li>'; the_category(' </li><li> '); if (is_single()) { echo "</li><li>"; the_title(); echo '</li>'; } } elseif (is_page()) { echo '<li>'; echo the_title(); echo '</li>'; } } elseif (is_tag()) {single_tag_title();} elseif (is_day()) {echo"<li>Archive for "; the_time('F jS, Y'); echo'</li>';} elseif (is_month()) {echo"<li>Archive for "; the_time('F, Y'); echo'</li>';} elseif (is_year()) {echo"<li>Archive for "; the_time('Y'); echo'</li>';} elseif (is_author()) {echo"<li>Author Archive"; echo'</li>';} elseif (isset($_GET['paged']) && !empty($_GET['paged'])) {echo "<li>Blog Archives"; echo'</li>';} elseif (is_search()) {echo"<li>Search Results"; echo'</li>';} echo '</ul>'; }
You can add this code to your single.php file or page.php to display the breadcrumbs menu.
<?php the_breadcrumb(); ?>
Note: If this is your first time adding code snippets in WordPress, then please refer to our guide on how to properly add code snippets in WordPress, so you don’t accidentally break your site.
If you liked this code snippet, please consider checking out our other articles on the site like: 9 best WordPress accordion plugins and how to create a contact form .
Hi
how can I include pages made from plugins I used the function dimox_breadcrumbs().
My plugin pages are from Give Donation and Newsletter plugin
Thanks this code really helps!
I have been researching on how to add a page to your breadcrumbs. Okay for example I have a page called Orange and a post category called orange for these are connected. How do you create a breadcrumb like this:
Home / Orange (page) / Title of the post (post)
They can then click on Orange page to view more post to read.
Each page I have wording. Then I add a post view of the current post for that topic of the page.
I want them to be able to go back to the home page where they found the current post.
Can you help me? Thank you for your time.
Excellent tutorial on how to implement a breadcrumb function instead of relying on plugins.. anyway, this can be improved a lot better by inserting structured data for breadcrumb..
This is awesome and very helpful. I added a few custom post types and this script breaks on those templates. Could you help with an addition for custom post types? Thank you!
Can you please help with a version that includes schema.org markups?
Thanks for this!
Just a quick note: you missed out {} in