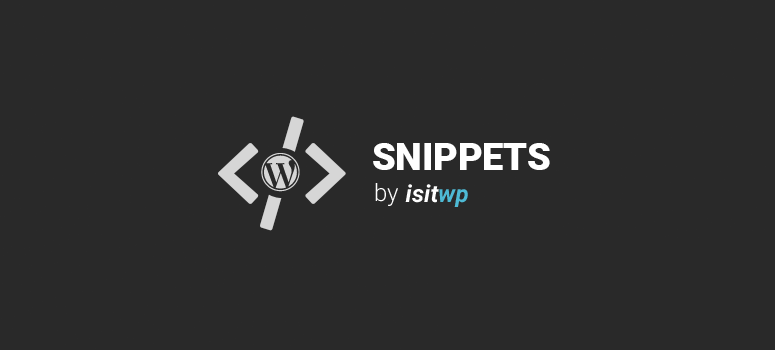
Do you want to add custom status message for each post that an author creates? While there’s probably a plugin for this, we have created a quick code snippet that you can use to create custom post status message in admin in WordPress.
Instructions:
All you have to do is add this code to your theme’s functions.php file or in a site-specific plugin.
Only users with the publish_posts
capability can change the status while everyone sees what the status is (if it is set). You can remove the status notification by selecting none.
add_filter( 'display_post_states', 'custom_post_state' ); function custom_post_state( $states ) { global $post; $show_custom_state = get_post_meta( $post->ID, '_status' ); // We are using "None" as a way to disable this feature for the current post. if ( $show_custom_state && $show_custom_state[0] != 'None' ) $states[] = '<span class="custom_state ' . strtolower( $show_custom_state[0] ) . '">' . $show_custom_state[0] . '</span>'; return $states; } add_action( 'admin_head', 'status_css' ); function status_css() { echo ' <!-- Styling of Custom Statuses --> <style type="text/css"> .custom{border-top:solid 1px #e5e5e5;} .custom_state{ font-size:9px; color:#666; background:#e5e5e5; padding:3px 6px 3px 6px; -moz-border-radius:3px; -webkit-border-radius:3px; border-radius:3px; border-radius:3px; } .spelling{background:#4BC8EB;color:#fff;} .review{background:#CB4BEB;color:#fff;} .errors{background:#FF0000;color:#fff;} .source{background:#D7E01F;color:#333;} .rejected{background:#000000;color:#fff;} .final{background:#DE9414;color:#333;} </style>'; } // Only those with the capability should be able to change things. if ( current_user_can( 'publish_posts' ) ) { // Insert our "Custom Status" into the Post Publish Box add_action( 'post_submitbox_misc_actions', 'custom_status_metabox' ); function custom_status_metabox() { global $post; $custom = get_post_custom( $post->ID ); $status = $custom["_status"][0]; $i = 0; // Available Statuses $custom_status = array( 'None', 'Spelling', 'Review', 'Errors', 'Source', 'Rejected', 'Final' ); echo ' <div class="misc-pub-section custom">Custom status: <select name="ourstatus">'; for ( $i = 0; $i < count( $custom_status ); $i++ ) { echo '<option value="' . $custom_status[$i] . '"'; if ( $status == $custom_status[$i] ) echo ' selected="selected"'; echo '>' . $custom_status[$i] . '</option>'; } echo '</select></div>'; } // Save add_action( 'save_post', 'save_status' ); function save_status( $post_id ) { if ( defined( 'DOING_AUTOSAVE' ) && DOING_AUTOSAVE ) return $post_id; update_post_meta( $post_id, "_status", $_POST["ourstatus"] ); } }
Note: If this is your first time adding code snippets in WordPress, then please refer to our guide on how to properly add code snippets in WordPress, so you don’t accidentally break your site.
If you liked this code snippet, please consider checking out our other articles on the site like: How to create stunning WordPress optin forms and 10 best WordPress testimonial plugins.
GREAT!!!!!!!!!!!!! Saved my life!
hi, is there any chance to hide this feature from non administrators in wordpress?
If you want to remove all aspects from regular users you could just do something like this with the hooks,
if(is_admin()){
add_action( ‘post_submitbox_misc_actions’, ‘custom_status_metabox’ );
add_filter( ‘display_post_states’,’custom_post_state’);
}
If you want to display the status but not give non admin users access to change it you can do the same but just with the function that displays the interface options.
if(is_admin()){
add_action( ‘post_submitbox_misc_actions’, ‘custom_status_metabox’ );
}
I have not tested this but should work,
below, not bellow. 🙂
WHooops 🙂 ty
[…] [Source: WPSNIPP] […]
[…] wordpress theme will let you create custom post status messages. This is an updated version of the (Create custom post status messages in admin) adding some additional features. Only users with the “publish_posts” capability can […]
Great addon 😉 I was wondering, i tried it on my WP 3.1.3 and it works great. The only thing i wanted to ask is how can i remove the status, after the article has been re-uploaded. I tried using the “Custom” or the “——” but than one of these show in the “Post” list as well.
The easy way would be to set display:none; on one of the statuses you create, eg: .final{display:none;} it would still create the status just not display it.
Thanks for this! I have adjusted your code to delete the status if set to “None”. Also included so only those who can “publish_posts” can change the status while everyone sees the saved results of course.
add_filter( ‘display_post_states’, ‘custom_post_state’ );
function custom_post_state( $states ) {
global $post;
$show_custom_state = get_post_meta( $post->ID, ‘_status’ );
if ( $show_custom_state ) $states[] = ” . $show_custom_state[0] . ”;
return $states;
}
add_action( ‘admin_head’, ‘status_css’ );
function status_css() {
echo ‘
.default{font-weight:bold;}
.custom{border-top:solid 1px #e5e5e5;}
.custom_state{font-size:9px; color:#666; background:#e5e5e5; padding:3px 6px 3px 6px; -moz-border-radius:3px; border-radius:3px;}
.spelling{background:#4BC8EB;color:#fff;}
.review{background:#CB4BEB;color:#fff;}
.errors{background:#FF0000;color:#fff;}
.source{background:#D7E01F;color:#333;}
.rejected{background:#000000;color:#fff;}
.final{background:#DE9414;color:#333;}
‘;
}
if ( current_user_can( ‘publish_posts’ ) ) {
add_action( ‘post_submitbox_misc_actions’, ‘custom_status_metabox’ );
add_action( ‘save_post’, ‘save_status’ );
function custom_status_metabox() {
global $post;
$custom = get_post_custom( $post->ID );
$status = $custom[“_status”][0];
$i = 0;
// Available Statuses
$custom_status = array( ‘None’, ‘Spelling’, ‘Review’, ‘Errors’, ‘Source’, ‘Rejected’, ‘Final’ );
echo ‘Custom status: ‘;
foreach ( $i = 0; $i < count( $custom_status ); $i++ ) {
echo '’ . $custom_status[$i] . ”;
}
echo ”;
}
function save_status( $post_id ) {
if ( defined( ‘DOING_AUTOSAVE’ ) && DOING_AUTOSAVE ) return $post_id;
// If the status is set to “None” we want to delete this setting.
if ( $_POST[“ourstatus”] == ‘None’ ) delete_post_meta( $post_id, “_status”, $_POST[“ourstatus”] );
else update_post_meta( $post_id, “_status”, $_POST[“ourstatus”] );
}
}
Cool Gabriel nice update, you should contact me via http://wpsnipp.com/contact/So I can post the update attributed to you as post author.
Made a slight modification to the code, yours had syntax errors and you used “foreach” instead of “for”
add_filter( ‘display_post_states’, ‘custom_post_state’ );
function custom_post_state( $states ) {
global $post;
$show_custom_state = get_post_meta( $post->ID, ‘_status’ );
if ( $show_custom_state ) $states[] = ” . $show_custom_state[0] . ”;
return $states;
}
add_action( ‘admin_head’, ‘status_css’ );
function status_css() {
echo ‘
.default{font-weight:bold;}
.custom{border-top:solid 1px #e5e5e5;}
.custom_state{font-size:9px; color:#666; background:#e5e5e5; padding:3px 6px 3px 6px; -moz-border-radius:3px; border-radius:3px;}
.spelling{background:#4BC8EB;color:#fff;}
.review{background:#CB4BEB;color:#fff;}
.errors{background:#FF0000;color:#fff;}
.source{background:#D7E01F;color:#333;}
.rejected{background:#000000;color:#fff;}
.final{background:#DE9414;color:#333;}
‘;
}
if ( current_user_can( ‘publish_posts’ ) ) {
add_action( ‘post_submitbox_misc_actions’, ‘custom_status_metabox’ );
add_action( ‘save_post’, ‘save_status’ );
function custom_status_metabox() {
global $post;
$custom = get_post_custom( $post->ID );
$status = $custom[“_status”][0];
$i = 0;
// Available Statuses
$custom_status = array( ‘None’, ‘Spelling’, ‘Review’, ‘Errors’, ‘Source’, ‘Rejected’, ‘Final’ );
echo ‘Custom status: ‘;
for ( $i = 0; $i < count( $custom_status ); $i++ ) {
echo '’ . $custom_status[$i] . ”;
}
echo ”;
}
function save_status( $post_id ) {
if ( defined( ‘DOING_AUTOSAVE’ ) && DOING_AUTOSAVE ) return $post_id;
// If the status is set to “None” we want to delete this setting.
if ( $_POST[“ourstatus”] == ‘None’ ) delete_post_meta( $post_id, “_status”, $_POST[“ourstatus”] );
else update_post_meta( $post_id, “_status”, $_POST[“ourstatus”] );
}
}
if ( $_POST[“ourstatus”] == ‘None’ ) delete_post_meta( $post_id, “_status”, $_POST[“ourstatus”] );
is throwing an error: Undefined index: ourstatus
So I did this:
if ( isset($_POST[“ourstatus”]) == ‘None’ ) delete_post_meta( $post_id, “_status”, $_POST[“ourstatus”] );
else update_post_meta( $post_id, “_status”, $_POST[“ourstatus”] );
is throwing the same error: Undefined index: ourstatus
so did the same:
if ( isset($_POST[“ourstatus”]) == ‘None’ ) delete_post_meta( $post_id, “_status”, isset($_POST[“ourstatus”] ));
But I can not seem to clear this final error:
Undefined index: ps_right_now in . . . wp-content/plugins/post-status-menu-items/cms_post_status_menu.php
Hi Antonin,
This is a very late response, but I wanted to let you know that I believe that last error you mentioned was fixed this morning in version 1.3.3 of Post Status Menu Items.
[…] is a great snipped wrote by Kevin Chard and applying this snippet to your function.php you can create cusom icon for custom post type; but […]